Oh noes!
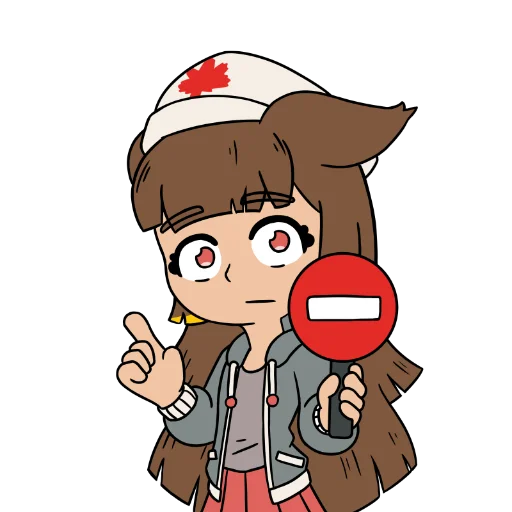
Access Denied: error code 9cbadf261a4db6284eb5ae6d3017640606e06bb67bfb1deea02548ef99ebc246.
Go home or if you believe you should not be blocked, please contact the webmaster at lionel@les-miquelots.net
Access Denied: error code 9cbadf261a4db6284eb5ae6d3017640606e06bb67bfb1deea02548ef99ebc246.
Go home or if you believe you should not be blocked, please contact the webmaster at lionel@les-miquelots.net